Vue JS is a progressive JavaScript framework that simplifies front-end development with its flexible and lightweight architecture. One of the core strengths of Vue JS is the Vue JS Component, which allows developers to build reusable and modular UI elements. These Vue JS Components help create scalable applications while improving code organization and maintainability.
Understanding the meaning of Vue is essential for developers looking to enhance their projects. Vue definition refers to its ability to provide a reactive and efficient development experience. Among the features of Vue, its component-based structure, virtual DOM, and reactive data binding stand out, making development smoother and more efficient.
By leveraging Vue JS Components, developers can create dynamic and interactive web applications with ease. Whether you’re exploring Vue JS for the first time or looking to optimize your existing project, mastering components is a key step in building high-quality applications.
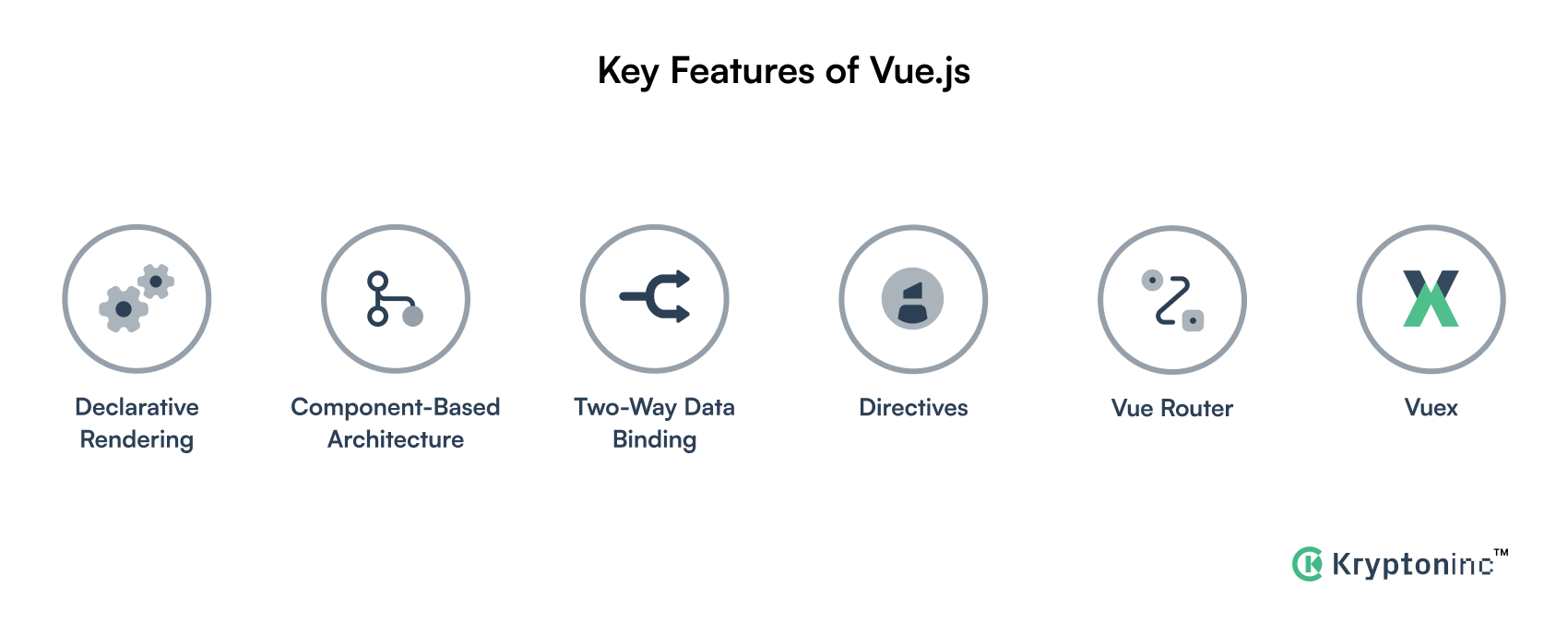
Meaning of Vue
Vue is a progressive JavaScript framework used for building dynamic user interfaces and single-page applications. It is designed to be lightweight, flexible, and easy to integrate. Vue’s reactive data-binding and component-based architecture make it a powerful choice for creating scalable, interactive web applications with a seamless user experience.
Vue Definition
Vue is a progressive JavaScript framework used for building modern web applications. It follows a component-based architecture, allowing developers to create reusable UI elements. With features like reactive data binding, a virtual DOM, and easy integration, Vue simplifies front-end development while enhancing performance, scalability, and user experience.
Explore our Custom Software Development services, designed to create tailored solutions that meet your unique business needs. We build scalable, secure, and high-performance software to streamline operations and drive growth.
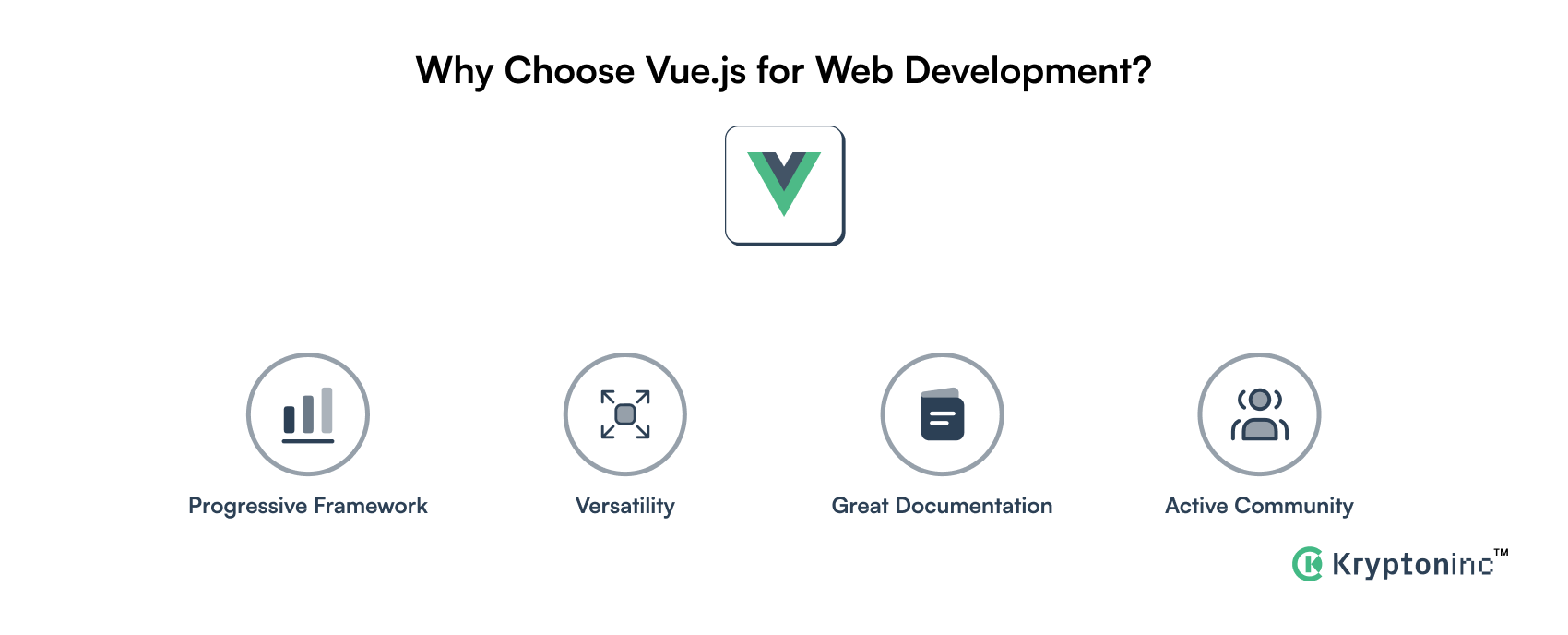
What is a Vue JS Component?
A Vue JS Component is a reusable piece of UI that encapsulates HTML, CSS, and JavaScript logic. Components help in breaking down complex user interfaces into smaller, manageable parts, improving both maintainability and reusability.
Example of a Simple Vue Component
Features of Vue
Vue JS is a progressive JavaScript framework designed for building dynamic user interfaces and single-page applications. It is widely recognized for its simplicity, flexibility, and efficiency. Below are the key features of Vue that make it a popular choice among developers.
1. Reactive Data Binding
Vue uses a reactive data-binding system, ensuring that the UI updates automatically whenever the data changes. This eliminates the need for manual DOM manipulation, making development faster and more efficient.
2. Virtual DOM
Vue utilizes a virtual DOM, which optimizes rendering performance. Instead of directly modifying the actual DOM, Vue first updates a virtual representation and then efficiently applies the necessary changes, improving speed and reducing rendering lag.
3. Component-Based Architecture
One of the core features of Vue is its component-based architecture, which allows developers to create reusable and modular components. This enhances code maintainability and scalability, making development more organized.
4. Directives
Vue provides built-in directives like v-if, v-for, and v-bind, which help developers manipulate the DOM easily. These directives add dynamic behavior to templates without writing extra JavaScript code.
5. Vue Router & State Management
Vue offers Vue Router for handling client-side navigation and Vuex for state management. These tools simplify the development of complex applications by managing routing and application state efficiently.
6. Computed Properties & Watchers
Vue includes computed properties and watchers, allowing developers to perform real-time calculations and track data changes without writing extra code.
7. Easy Integration & Flexibility
Vue can be easily integrated into existing projects and works well with other libraries. Its incrementally adaptable nature makes it ideal for small projects as well as large-scale applications.
With these powerful features, Vue JS remains a top choice for developers looking to build scalable, efficient, and high-performance web applications.
Key Features of Vue Components
Vue components are a fundamental part of Vue JS, allowing developers to build modular, reusable, and scalable applications. Below are the key features of Vue components that make them essential for efficient development.
1. Encapsulation
Each Vue component is self-contained, meaning it has its own logic, styles, and structure. This encapsulation ensures that changes in one component do not affect others, leading to better code organization and reduced conflicts in large projects.
2. Reusability
One of the biggest advantages of Vue components is their reusability. A single component can be used multiple times across an application, reducing redundant code and speeding up development. This feature makes Vue highly efficient for building UI elements like buttons, forms, and modals.
3. Maintainability
By breaking down the UI into smaller Vue components, developers improve code readability and maintenance. Instead of working with a large monolithic codebase, they can manage individual components separately, making debugging and updates easier.
4. Scalability
Vue components provide a scalable architecture, allowing developers to build complex applications by combining multiple small, modular components. This approach ensures that as the application grows, it remains maintainable and efficient.
With these powerful features, Vue components enable developers to create dynamic, high-performance web applications while keeping the code clean and manageable.
Benefits of Using Vue JS Components
Vue JS components offer numerous advantages, making web development more efficient, maintainable, and scalable. Here are some key benefits of using Vue JS components:
1. Modularity
Vue components enable modular development by breaking down complex UIs into small, reusable parts. This makes the codebase more organized and easier to manage, especially in large applications.
2. Improved Performance
Vue’s reactive data-binding system ensures that components update only when necessary, optimizing performance. Instead of re-rendering the entire DOM, Vue efficiently updates only the affected components, leading to faster page loads and smoother interactions.
3. Easier Debugging
Since components are self-contained, developers can isolate and troubleshoot code efficiently. Debugging becomes easier as errors are confined to specific components rather than affecting the entire application.
4. Code Reusability
Vue JS components promote code reusability, reducing redundancy and improving maintainability. Developers can reuse components across different parts of the application, making development more efficient.
5. Faster Development
By leveraging pre-built and reusable components, developers can significantly speed up project timelines. This accelerates development while maintaining consistency across the application.
With these benefits, Vue JS components streamline development, enhance performance, and improve code maintainability, making them an essential part of modern web applications.
Types of Vue JS Components
Vue components can be categorized based on their usage and scope.
1. Global Components
A global component is registered once and can be used anywhere in the app.
✅ Example of a Global Component
import { createApp } from 'vue';
import ButtonComponent from './ButtonComponent.vue';
const app = createApp(App);
app.component('ButtonComponent', ButtonComponent);
app.mount('#app');
2. Local Components
A local component is registered within a specific component and is accessible only inside that component.
✅ Example of a Local Component
📌 When to Use:
🔹 Use global components for common UI elements like buttons, modals, or alerts.
🔹 Use local components for context-specific features like form inputs or sidebars.
How to Create and Use Vue Components
Step 1: Create a Vue Component
A component file has a .vue extension and consists of three sections:
✅ Example of a Vue Component (Card.vue)
{{ title }}
{{ content }}
Step 2: Use the Component
Vue Props, Events, and Slots for Dynamic Components
1. Props: Passing Data to a Child Component
Props allow data to flow from a parent component to a child component.
✅ Example: Using Props
Hello, {{ name }}!
2. Events: Communicating from Child to Parent
Use the $emit method to send events from a child component to its parent.
✅ Example: Button Component with Event Emission
Usage in Parent Component:
3. Slots: Creating Flexible Components
Slots allow components to accept dynamic content.
✅ Example: Creating a Card Component with a Slot
Usage with Custom Content:
Custom Title
This content is passed via a slot.
Best Practices for Reusable Vue JS Components
1. Keep Components Small and Focused
Each component should serve a single purpose.
2. Use Props and Events for Communication
Avoid tightly coupling components by using props and events.
3. Optimize Rendering with Computed Properties
Use computed properties instead of modifying the DOM directly.
4. Avoid Side Effects in Components
Keep business logic separate from UI logic by using Vuex or Pinia.
5. Use Scoped Styles
Use the scoped attribute to prevent styles from affecting other components.
Testing Vue Components for Maintainability
Testing Vue JS components is essential for ensuring maintainability, performance, and reliability in applications. Well-tested components help developers catch bugs early, improve code quality, and simplify future updates.
Why Test Vue Components?
✅ Ensures Stability – Detects issues before deployment.
✅ Improves Maintainability – Simplifies debugging and refactoring.
✅ Enhances Performance – Prevents memory leaks and inefficiencies.
✅ Facilitates Collaboration – Helps teams work on shared components confidently.
Types of Tests for Vue Components
- Unit Tests – Test isolated component logic.
- Integration Tests – Verify interactions between components.
- End-to-End (E2E) Tests – Simulate real user interactions.
Unit Testing Vue Components with Jest & Vue Test Utils
Unit tests check if individual Vue components work as expected. The Vue Test Utils library provides utilities to test Vue components efficiently.
✅ Example: Testing a Button Component
import { mount } from '@vue/test-utils';
import Button from '@/components/Button.vue';
test('renders button with correct text', () => {
const wrapper = mount(Button, {
props: { label: 'Click Me' }
});
expect(wrapper.text()).toBe('Click Me');
});
✅ Example: Testing Click Event Emission
test('emits event when clicked', async () => {
const wrapper = mount(Button);
await wrapper.trigger('click');
expect(wrapper.emitted()).toHaveProperty('click');
});
Best Practices for Testing Vue Components
🔹 Write small, focused tests for each component.
🔹 Use mock data and dependencies to isolate behavior.
🔹 Test props, computed properties, and event emissions.
🔹 Run tests automatically in CI/CD pipelines for consistent quality.
By implementing proper testing strategies, developers can maintain scalable and bug-free Vue applications.
Conclusion
Vue JS Components are essential for building scalable and maintainable applications. By following best practices, developers can create reusable, efficient, and performance-optimized components. Whether you’re a beginner or an experienced developer, mastering Vue components will improve your development process and application architecture.
📌 Key Takeaways:
✅ Vue components improve code reusability and maintainability.
✅ Use props, events, and slots for flexible and reusable components.
✅ Follow best practices to enhance performance and scalability.
✅ Test components using Jest and Vue Test Utils.
Looking for expert Vue.js development services? Our team specializes in building high-performance, scalable applications using Vue JS Components. Whether you need a custom solution, component optimization, or full-stack development, we’re here to help!